Gain essential web programming skills
Learn one of the three core web technologies used to create interactive web pages
JavaScript is a powerful scripting language which can be used to read and modify HTML elements, validate data, and it can also react to events such as a mouse click or a key press on the keyboard, etc.
A majority of website on the internet use JavaScript in some way, so it is one of the must have skills for a programmer or web developer
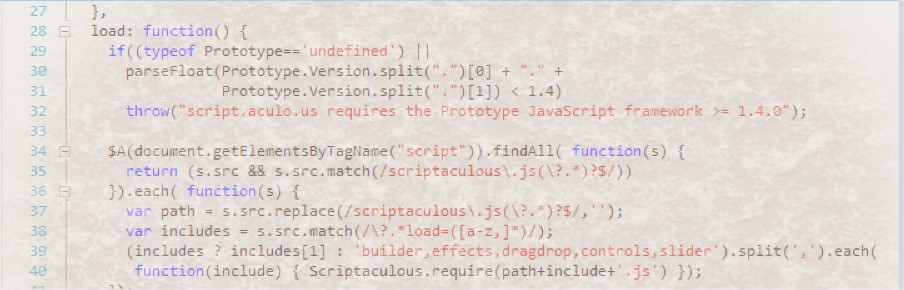
Create event driven, functional and imperative web pages, JavaScript provides powerful abilities to the developer
Easy to learn, this course will give novices an understanding of how to use JavaScript with HTML to create web pages
Interested in JavaScript; look at our HTML, ASP.net, VB.net & SQL courses to make dynamic responsive websites