Learn to make Windows Programs using .NET (Visual Basic)
Learn Microsoft development Language. This Language helps with ASP.NET projects as they can now use Visual Basic.Net code in the background for website functionality.
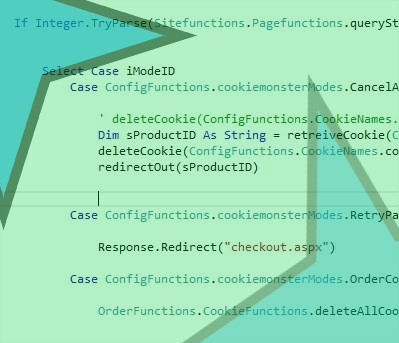
History of BASIC
By the mid-1970s, as personal computers became cheaper and more widely available, people started owning their own, but most people found they were still hard to program. In response to this need, Bill Gates and Paul Allen produced a version of BASIC and started selling it from their newly formed company, Microsoft. Software built on the .NET Framework can be easier to deploy and maintain than conventional software. Applications can be designed to automatically upgrade themselves to the latest version. The .NET Framework can also minimise conflicts between applications by helping incompatible software components coexist.